In today’s world, how well your web apps perform is crucial. For React apps, making sure they run smoothly is essential. As your application grows, even small issues can cause slowdowns, longer load times, and a drop in performance, and a slow or laggy app can upset users, make them leave quickly, and hurt your search engine rankings.
React is known for its efficiency, but even the best frameworks can struggle with performance issues, especially when handling large amounts of data, complex components, or frequent updates.
In this post, we’ll go over easy-to-follow tips and tricks to optimize your React app’s performance. You’ll learn how to spot and fix common problems so your app can run faster and keep your users happy.
What Affects Performance in React Applications?
Wondering why your React app isn’t running as smoothly as you’d like? Understanding what affects performance can be a game-changer. But before we explore what affects your app’s speed, let’s first look at the key metrics you need to know.
Explanation of Key Performance Metrics
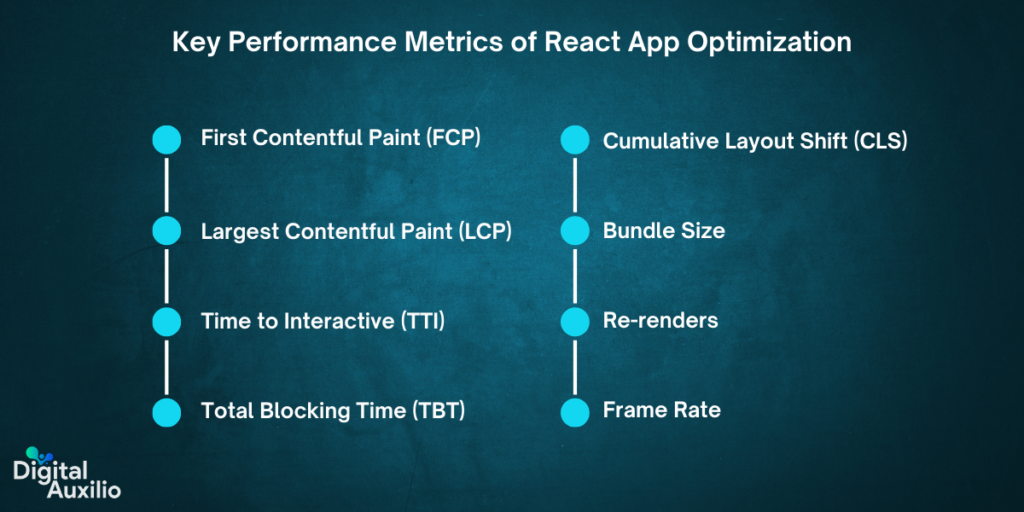
To understand what affects performance in React applications, it’s crucial to be familiar with key performance metrics that help gauge how well an application is performing:
- First Contentful Paint (FCP): This measures the time it takes for the first piece of content (text or image) to be rendered on the screen. A low FCP indicates that users can see and interact with content quickly, improving their first impression of the app.
- Largest Contentful Paint (LCP): LCP measures the time it takes for the largest content element (usually an image or a block of text) to become visible within the viewport. This metric is crucial for understanding how quickly the main content of your app is loaded.
- Time to Interactive (TTI): The time it takes for the application to become fully interactive, allowing users to interact with all functionalities without delays. It includes considerations of JavaScript execution and network latency.
- Total Blocking Time (TBT): TBT assesses the total time during which the main thread is blocked and cannot respond to user interactions. Reducing TBT is important for improving the app’s responsiveness.
- Cumulative Layout Shift (CLS): CLS measures the visual stability of the page by tracking unexpected layout shifts that occur during page load. A lower CLS value means fewer shifts, leading to a more stable and user-friendly experience.
- Bundle Size: This metric refers to the size of the JavaScript bundles that need to be downloaded and parsed by the browser. Smaller bundle sizes usually result in faster load times and improved performance.
- Re-renders: This metric tracks how often React components are re-rendered. Excessive re-renders can degrade performance by causing unnecessary calculations and DOM updates.
- Frame Rate: Frame rate, or frames per second (FPS), reflects how smoothly animations and transitions run. A higher frame rate (e.g., 60 FPS) results in smoother animations. Performance can be improved by avoiding heavy computations during animation and leveraging requestAnimationFrame.
Common Performance Bottlenecks in React Apps
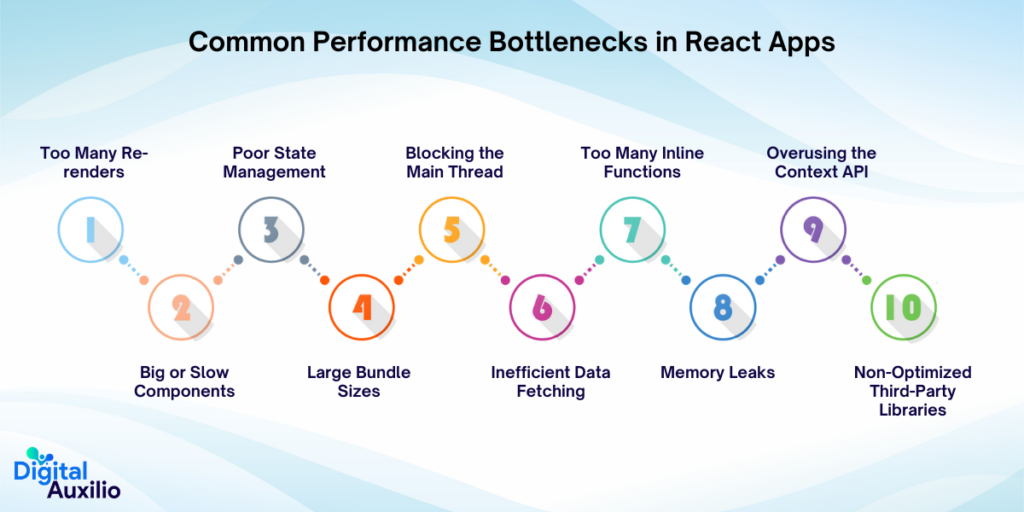
When developing React applications, performance can be a significant concern. Identifying and addressing performance bottlenecks is crucial to ensure a smooth and responsive user experience. Here are some common performance bottlenecks in React apps:
- Too Many Re-renders: React components can re-render more than needed, which can make the app slower. This often happens if the state or props change too often or are not managed well.
- Big or Slow Components: Components that are too big or complicated can take a long time to render. If they aren’t optimized, they can slow down the app.
- Poor State Management: If the state is not managed properly, it can cause performance problems. Frequent or unnecessary state updates can make the app less responsive.
- Large Bundle Sizes: If the JavaScript bundle is too big, the app will take longer to load. A large bundle means it takes more time to download and run the code.
- Blocking the Main Thread: Long tasks that block the main thread can make the app feel slow. If the main thread is busy, it delays rendering and user interactions.
- Inefficient Data Fetching: Fetching too much data or doing it too often can slow down the app. Good data-fetching strategies are important for keeping the app responsive.
- Too Many Inline Functions: Using inline functions in render methods can cause performance issues. These functions can lead to unnecessary re-renders.
- Memory Leaks: Memory leaks happen when resources or event listeners are not properly cleaned up. This can use up more memory and slow down the app over time.
- Overusing the Context API: Using the Context API too much or incorrectly can cause performance problems. It can trigger extra re-renders if not used carefully.
- Non-Optimized Third-Party Libraries: Some third-party libraries might not be efficient and can affect performance. It’s important to choose well-maintained and optimized libraries.
Read Also – React vs React Native: Which Framework Reigns Supreme?
Proven Ways to Improve React Performance
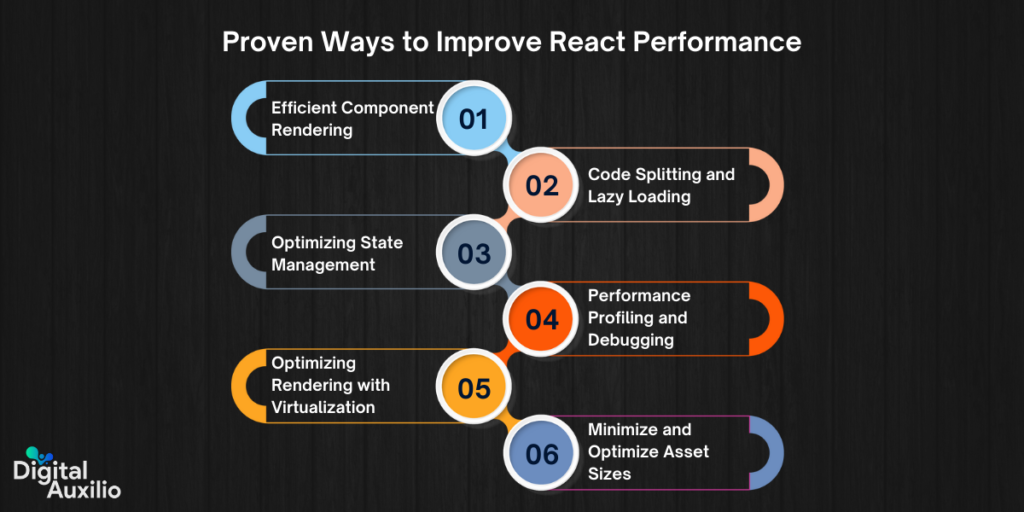
React performance optimization is crucial for creating smooth and responsive user experiences. By optimizing how your React application functions, you can achieve faster load times, better user interactions, and a more efficient overall experience. Here are proven methods to enhance the performance of your React applications:
1. Efficient Component Rendering:
To make React apps faster, avoid unnecessary re-renders. Use React.memo to keep components from re-rendering if their props haven’t changed. Also, use shouldComponentUpdate or PureComponent to stop re-renders when there’s no need. For functions and values that take a lot of time to compute, use useMemo and useCallback hooks to store their results and avoid recalculating them.
Use React.memo to Prevent Unnecessary Re-renders
How React.memo Works?
React.memo is a higher-order component that optimizes performance by memoizing a component. This means React will only re-render the component if its props have changed. It’s particularly useful for functional components that render the same output given the same input, making it an efficient way to prevent unnecessary re-renders.
Examples of Where to Apply React.memo
- Static Lists: If you have a list component that displays static items and doesn’t change frequently, wrapping it in React.memo will prevent re-renders when parent components update.
- Expensive Components: For components that perform expensive calculations or render complex UI, React.memo can help avoid unnecessary updates, improving overall performance.
Code Example:
const ListItem = React.memo(({ item }) => { console.log(‘Rendering:’, item.name); return <li>{item.name}</li>; }); |
Optimize Functional Components with useCallback and useMemo
Differences Between useCallback and useMemo:
- useCallback: This hook memoizes callback functions, ensuring they remain the same between renders unless their dependencies change. It’s useful for passing stable callback functions to child components, preventing them from re-rendering if the function reference remains the same.
- useMemo: This hook memoizes the result of a computation, only recalculating it when its dependencies change. It’s useful for optimizing expensive calculations or derived data, ensuring they don’t get recalculated on every render.
Practical Examples and Use Cases:
- useCallback Example: If you pass a callback function to a child component, wrapping it with useCallback can prevent unnecessary re-renders of that child component.
const handleClick = useCallback(() => { console.log(‘Button clicked’); }, []); |
- useMemo Example: If you have a function that calculates a derived value from props or state, useMemo can cache the result of this calculation.
const expensiveCalculation = useMemo(() => { return computeExpensiveValue(data); }, [data]); |
Avoid Inline Functions and Anonymous Functions
Impact on Performance:
Inline and anonymous functions can lead to performance issues because they are recreated on every render. This can cause unnecessary re-renders of child components, as their props are perceived as changed due to the new function reference.
Best Practices for Function Definition:
- Define Functions Outside of Render: Move functions out of the render method or functional component body. Define them at the top level or outside the component if possible.
- Use useCallback for Handlers: Wrap event handlers or other functions with useCallback to memoize them and prevent re-creation on every render.
- Code Example:
// Avoid this const Component = () => { return <button onClick={() => console.log(‘Clicked’)}>Click Me</button>; }; // Prefer this const handleClick = () => { console.log(‘Clicked’); }; const Component = () => { return <button onClick={handleClick}>Click Me</button>; }; |
1. Import React.lazy and Suspense:
import React, { Suspense } from ‘react’; |
2. Define a Component Using React.lazy:
const MyComponent = React.lazy(() => import(‘./MyComponent’)); |
This tells React to load MyComponent only when it’s needed.
3. Wrap the Lazy Component with Suspense:
function App() { return ( <Suspense fallback={<div>Loading…</div>}> <MyComponent /> </Suspense> ); } |
The fallback prop specifies what should be displayed while the component is loading.
Dynamic Imports for Better Load Times
How Dynamic Imports Work?
Dynamic imports allow you to load modules only when they are needed. This is done using the import() function, which returns a promise that resolves to the module.
Integration with Webpack and Other Bundlers
Webpack:
Webpack supports dynamic imports out of the box. It automatically splits your code into chunks and loads them as needed.
// Using dynamic import import(‘./module’).then((module) => { // Use the module here }); |
Other Bundlers:
Most modern bundlers, like Parcel and Rollup, also support dynamic imports and can handle code splitting similarly to Webpack.
3. Optimizing State Management
Keep state updates to a minimum and make sure they are efficient. Use React’s Context API or libraries like Redux to manage state, but try to keep state changes local. This helps avoid unnecessary re-renders and speeds up your app.
Best Practices for State Management in React
Use React’s Built-in State:
- Local State: For simple cases where you need to track information specific to a single component, React’s useState hook is usually enough.
- Advantages: It’s straightforward and keeps the component self-contained.
External State Management Libraries:
- When to Use: For complex applications with many components needing to share state or for more advanced state management needs, libraries like Redux or Zustand can help.
- Advantages: These libraries offer more control and advanced features like global state management and middleware for handling side effects.
Efficient Use of Context AP
When to Use Context API?
For Global Data: Use the Context API when you need to pass data through many layers of your component tree without prop drilling.
Examples: Theme settings, user authentication, or locale information.
Avoiding Performance Pitfalls with Context API:
Minimize Re-Renders: The Context API can cause all consuming components to re-render whenever the context value changes. To avoid this, you can:
- Use Memoization: Wrap your context value in useMemo to prevent unnecessary recalculations.
- Break Down Context: If you have different parts of state, consider splitting them into separate contexts to reduce re-renders.
- Optimize Components: Use React.memo to avoid unnecessary re-renders in components that consume context.
Summary
- For simple state: Use React’s built-in state.
- For complex state management: Consider external libraries.
- For global data: Use the Context API, but be mindful of its performance implications by memoizing values and avoiding excessive context usage.
4. Performance Profiling and Debugging
To find and fix performance issues, use React DevTools to check how your components are performing. Look at which components are re-rendering too often and see where you can make improvements. The React Profiler tool can help you see which parts of your app need work.
Using React DevTools for Performance Profiling
Overview of React DevTools features:
React DevTools is a powerful tool that allows developers to inspect their React components, check the state and props, and measure the performance of their application. Key features include:
- Component Tree: Visualize the component structure and see how components are nested.
- Profiler Tab: Record performance during specific interactions to analyze re-renders, component mounts, and unmounts.
- Highlight Updates: Visually see which components are re-rendering as the app runs.
How to analyze and interpret performance data:
Once you’ve recorded a profiling session using React DevTools, you’ll receive a timeline of component renders. Key data points to analyze include:
- Render Times: Identify components that take longer to render and investigate why.
- Re-renders: Look for unnecessary re-renders and consider using React.memo to prevent them.
- Wasted Renders: Focus on renders that do not contribute to UI updates, indicating potential areas for optimization.
Identifying and Fixing Performance Issues
Common performance issues and how to address them:
- Excessive Re-Renders: Components that re-render too often can slow down your app. To address this, use React.memo or PureComponent to prevent re-renders when props and state haven’t changed.
- Inefficient State Management: Large or deep component trees that depend on a single piece of state can cause unnecessary renders. Consider breaking down state into smaller, more manageable pieces using React’s Context API or state management libraries like Redux.
- Heavy Computations in Render: Avoid performing heavy calculations directly within render methods. Instead, use useMemo to memoize expensive computations and prevent them from re-running on every render.
Example scenarios and solutions:
- Scenario: You notice a component re-renders every time the parent re-renders, even though its props haven’t changed. Solution: Implement React.memo for that component to ensure it only re-renders when its props actually change.
- Scenario: A large list of items is being rendered, causing slow performance. Solution: Use React’s virtualization libraries like react-window to only render items currently visible on the screen, improving performance.
5. Optimizing Rendering with Virtualization
Virtualization improves performance by only rendering the items you can see on the screen. Libraries like react-window or react-virtualized help with this by only showing a few items at a time. This reduces the number of elements in the DOM and makes rendering faster.
What is Virtualization?
Virtualization in the context of React refers to the technique of rendering only the visible part of a list or grid on the screen, instead of rendering the entire list or dataset. This approach helps in improving the performance of a React application, especially when dealing with large datasets.
Benefits of Virtualization in Rendering
In a traditional rendering approach, React renders every single item in a list or grid, regardless of whether it’s currently visible on the screen. This can lead to performance issues like slow rendering times and increased memory usage, especially with large datasets. Virtualization solves this problem by only rendering the elements that are currently visible in the viewport, plus a small buffer of items just outside the viewport to handle scrolling.
Here are the benefits of virtualization:
- Improved Performance: By rendering only a subset of the items, the application uses less memory and renders faster.
- Reduced CPU Load: Fewer components mean less processing for updates and re-renders, which reduces the overall CPU load.
- Smoother User Experience: Users experience smoother scrolling and quicker load times as the app doesn’t lag due to rendering large lists.
Implementing Virtualized Lists with Libraries
To implement virtualization in React, you can use specialized libraries designed to handle the efficient rendering of large lists.
Overview of Popular Libraries
- react-window: A lightweight library that provides efficient virtualized list and grid rendering. It’s suitable for most use cases where simple virtualization is needed.
- react-virtualized: A more comprehensive library that offers a wider range of features like virtualized tables, lists, grids, and more. It’s ideal for complex scenarios where additional control over rendering is required.
Example Implementation and Benefits
Here’s a simple example of how you might use react-window to create a virtualized list:
import React from ‘react’; import { FixedSizeList as List } from ‘react-window’; const Row = ({ index, style }) => ( <div style={style}> Row {index} </div> ); const MyList = () => ( <List height={150} // height of the viewport itemCount={1000} // total number of items in the list itemSize={35} // height of each item width={300} // width of the list > {Row} </List> ); |
Benefits of this Implementation:
- Scalability: The app can handle thousands of items without a significant impact on performance.
- Resource Efficiency: Memory and processing power are conserved because only the visible portion of the list is rendered.
- Ease of Use: These libraries abstract away the complexities of virtualization, making it easy to implement and manage.
6. Minimize and Optimize Asset Sizes
Make your app load faster by reducing the size of your files. Minify and compress JavaScript, CSS, and images using tools like Webpack. Optimize images by using formats like WebP and make sure they adjust to different screen sizes. Use caching and CDNs to deliver assets quickly.
Image Optimization Techniques
Images are often the largest assets on a webpage, so optimizing them can lead to significant performance gains.
Best practices for image formats and sizes
- Choose the Right Format: Use appropriate image formats depending on the content. For example, use JPEG for photographs and PNG for images with transparency. Modern formats like WebP offer better compression and quality.
- Responsive Images: Serve different image sizes based on the device’s screen size. This can be done using the srcset attribute in HTML, allowing the browser to choose the most appropriate image size.
- Compression: Compress images to reduce file size without significantly affecting quality. Tools like TinyPNG or ImageOptim can help with this.
Tools for optimizing images
- ImageMagick: A powerful tool for processing and optimizing images. It supports various formats and can be used to batch-optimize images.
- Webpack Plugins: Tools like image-webpack-loader can be integrated into your build process to automatically optimize images during the bundling process.
- CDNs: Content Delivery Networks (CDNs) like Cloudflare or Imgix offer built-in image optimization features, serving images in optimal formats and sizes based on the user’s device.
Efficient Use of Fonts and Icons
Fonts and icons can add to the overall load time if not managed properly. Optimizing their use can lead to better performance.
Techniques for Font Loading and Icon Management
1. Font Loading:
- Subset Fonts: Only include the characters needed for your site instead of loading the entire font family. Tools like Google Fonts offer options to customize the subset.
- Asynchronous Loading: Use font-display: swap in CSS to load fonts asynchronously. This ensures that text remains visible during the font loading process, improving perceived performance.
- Preload Key Fonts: Use <link rel=”preload”> in HTML to load critical fonts earlier in the page load process. This can reduce the time it takes for fonts to appear on the screen.
2. Icon Management:
- SVG Icons: Use SVG (Scalable Vector Graphics) for icons as they are lightweight and scalable without losing quality. SVGs can be embedded directly into HTML, reducing HTTP requests.
- Icon Fonts: If using icon fonts like FontAwesome, consider using a subset of icons rather than loading the entire font library.
- Icon Sprites: Combine multiple icons into a single sprite sheet, reducing the number of HTTP requests and improving load times.
In Conclusion
Making your React app faster is really important for a great user experience. You can improve performance by using techniques like memoization, lazy loading, and code splitting. These methods help your app run more smoothly and quickly.
We encourage you to put these tips into practice and see the difference for yourself. If you have any additional tips or questions, feel free to share them in the comments below!
Looking to take your React.js app to the next level? Contact us for SEO-optimized React.js app development. Let’s work together to create a high-performing react app that stands out!
Add comment